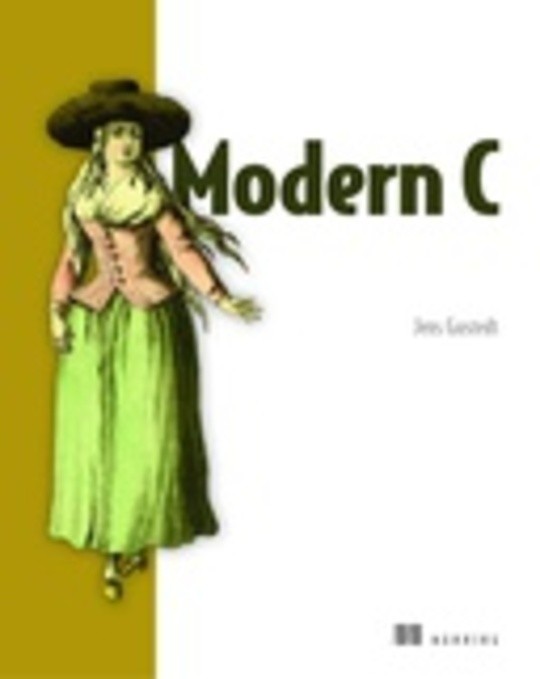
Modern C
Free
Description
Contents
Reviews
Language
English
ISBN
Unknown
About this book
C versions
C and C++
Requirements
Source code
Exercises and challenges
Organization
Author
Level 0. Encounter
1. Getting started
1.1. Imperative programming
1.2. Compiling and running
Summary
2. The principal structure of a program
2.1. Grammar
2.2. Declarations
2.3. Definitions
2.4. Statements
Summary
Level 1. Acquaintance
Buckle up
3. Everything is about control
3.1. Conditional execution
3.2. Iterations
3.3. Multiple selection
Summary
4. Expressing computations
4.1. Arithmetic
4.2. Operators that modify objects
4.3. Boolean context
4.4. The ternary or conditional operator
4.5. Evaluation order
Summary
5. Basic values and data
5.1. The abstract state machine
5.2. Basic types
5.3. Specifying values
5.4. Implicit conversions
5.5. Initializers
5.6. Named constants
5.7. Binary representions
Summary
6. Derived data types
6.1. Arrays
6.2. Pointers as opaque types
6.3. Structures
6.4. New names for types: type aliases
Summary
7. Functions
7.1. Simple functions
7.2. main is special
7.3. Recursion
Summary
8. C library functions
8.1. General properties of the C library and its functions
8.2. Mathematics
8.3. Input, output, and file manipulation
8.4. String processing and conversion
8.5. Time
8.6. Runtime environment settings
8.7. Program termination and assertions
Summary
Level 2. Cognition
9. Style
9.1. Formatting
9.2. Naming
Summary
10. Organization and documentation
10.1. Interface documentation
10.2. Implementation
Summary
11. Pointers
11.1. Pointer operations
11.2. Pointers and structures
11.3. Pointers and arrays
11.4. Function pointers
Summary
12. The C memory model
12.1. A uniform memory model
12.2. Unions
12.3. Memory and state
12.4. Pointers to unspecific objects
12.5. Explicit conversions
12.6. Effective types
12.7. Alignment
Summary
13. Storage
13.1. malloc and friends
13.2. Storage duration, lifetime, and visibility
13.3. Digression: using objects "before" their definition
13.4. Initialization
13.5. Digression: a machine model
Summary
14. More involved processing and IO
14.1. Text processing
14.2. Formatted input
14.3. Extended character sets
14.4. Binary streams
14.5. Error checking and cleanup
Summary
Level 3. Experience
15. Performance
15.1. Inline functions
15.2. Using restrict qualifiers
15.3. Measurement and inspection
Summary
16. Function-like macros
16.1. How function-like macros work
16.2. Argument checking
16.3. Accessing the calling context
16.4. Default arguments
16.5. Variable-length argument lists
16.6. Type-generic programming
Summary
17. Variations in control flow
17.1. A complicated example
17.2. Sequencing
17.3. Short jumps
17.4. Functions
17.5. Long jumps
17.6. Signal handlers
Summary
18. Threads
18.1. Simple inter-thread control
18.2. Race-free initialization and destruction
18.3. Thread-local data
18.4. Critical data and critical sections
18.5. Communicating through condition variables
18.6. More sophisticated thread management
Summary
19. Atomic access and memory consistency
19.1. The ``happened before'' relation
19.2. C library calls that provide synchronization
19.3. Sequential consistency
19.4. Other consistency models
Summary
Takeaways
Bibliography
Index
The book hasn't received reviews yet.