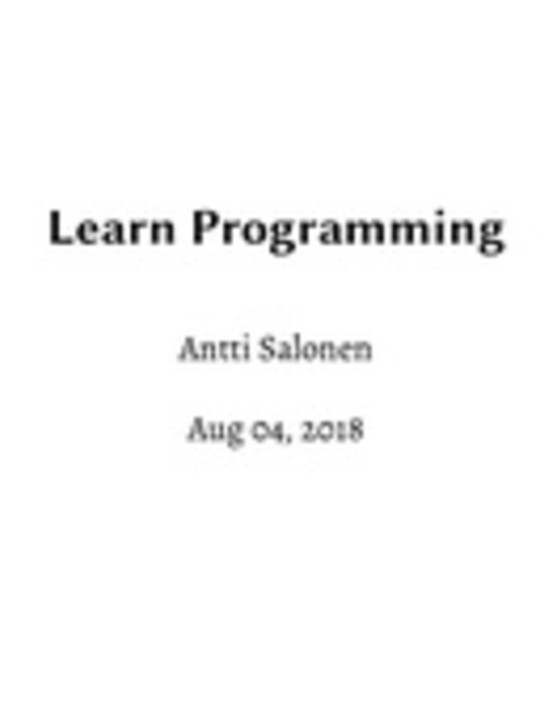
Learn Programming
Free
Description
Contents
Reviews
Language
English
ISBN
Unknown
The beginning
Introduction
Why this book?
What is software?
How does a computer work?
OK, but seriously, how does a computer work?
The basics of programming
Setting up the C toolchain
The basics of programming in C
Learning to learn
Basics of programming in Python and C
Quadratic formula in C
Lots of quadratic equations
Quadratic formula in Python
Generating input data using Python
Unix shell
Basic Unix shell usage
Unix shell scripting
Regular expressions
Using libraries in Python
Creating a simple web page
Making our web page work
Stage 1
Further Unix tools
Version control
Working with other git repositories
Some related Unix tools
Vim
Programming with vim
Background on programming languages and algorithms
Typing
Big O notation
Array, stack, queue
JavaScript
Guessing game in JS
JavaScript meets algorithms
Intermediate C
Records
C and the stack
Arrays and the stack
Dynamic memory allocation
C and strings
Writing a toy web server
Security
More programming concepts using Python
More data structures
Object oriented programming
JSON
More useful Python constructs
Callbacks and anonymous functions
Functional programming
Stage 1.5
Web development with Python and JavaScript
HTML tables
Redis
High level architecture
AJAX
Gluing AJAX and Redis together
The page for starting a new game
Generating the high score table
Some more tips and tricks
Working with binary data in C
PNG files
Finishing our simple PNG parser
Strongly, statically typed languages
Under the hood
Virtual machines
The case for statically, strongly typed languages
Established languages
Newer languages
Learning C++ using Sudoku
Introduction to Sudoku
Containers for Sudoku
Sudoku Puzzle class
Propagation and search
Stage 2
Larger software
Introduction to larger software
Breaking software down to components
Drawing to a screen using SDL2
Drawing the schedule screen using SDL2
Unix way - sched
Unix way - parse_gps
Unix way - merge
Monolithic way - parsing
Monolithic way - scheduled arrivals and GPS data
Monolithic way - merging and putting it all together
A fistful of Python exercises
Graphs
Parsing
SQL and its relationship with online shops
Introduction to SQL
Adding data to an SQL database
Querying SQL databases
Generating a return form
Web UI for our return form
Final bits
Software licenses
NP-hard problems
Concurrency
Tech behind this book
Further reading
Chapter dependencies
The book hasn't received reviews yet.