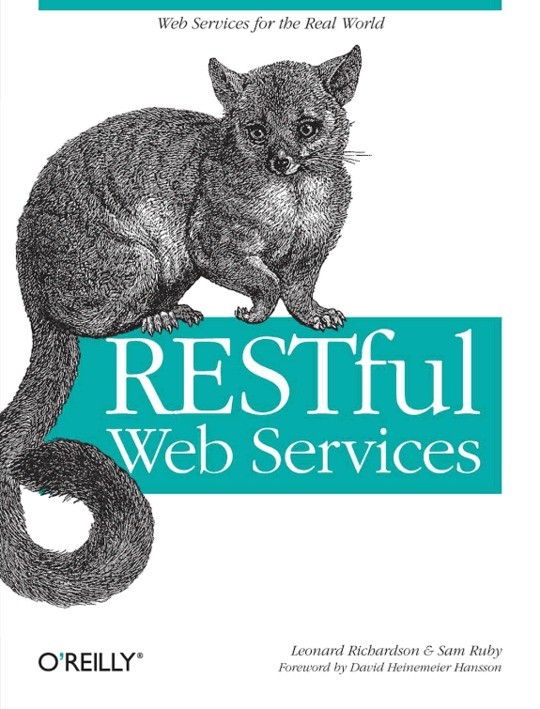
RESTful web services
Free
Description
Contents
Reviews
Language
English
ISBN
978-0-596-52926-0
Table of Contents
Foreword
Preface
The Web Is Simple
Big Web Services Are Not Simple
The Story of the REST
Reuniting the Webs
What’s in This Book?
Administrative Notes
Conventions Used in This Book
Using Code Examples
Safari® Enabled
How to Contact Us
Acknowledgments
Chapter 1. The Programmable Web and Its Inhabitants
Kinds of Things on the Programmable Web
HTTP: Documents in Envelopes
Method Information
Scoping Information
The Competing Architectures
RESTful, Resource-Oriented Architectures
RPC-Style Architectures
REST-RPC Hybrid Architectures
The Human Web Is on the Programmable Web
Technologies on the Programmable Web
HTTP
URI
XML-RPC
SOAP
WS-*
WSDL
WADL
Leftover Terminology
Chapter 2. Writing Web Service Clients
Web Services Are Web Sites
Wrappers, WADL, and ActiveResource
del.icio.us: The Sample Application
What the Sample Clients Do
Making the Request: HTTP Libraries
Optional Features
Ruby: rest-open-uri and net/http
Python: httplib2
Java: HttpClient
C#: System.Web.HTTPWebRequest
PHP: libcurl
JavaScript: XMLHttpRequest
The Command Line: curl
Other Languages
Processing the Response: XML Parsers
Ruby: REXML, I Guess
Python: ElementTree
Java: javax.xml, Xerces, or XMLPull
C#: System.Xml.XmlReader
PHP
JavaScript: responseXML
Other Languages
JSON Parsers: Handling Serialized Data
Clients Made Easy with WADL
Chapter 3. What Makes RESTful Services Different?
Introducing the Simple Storage Service
Object-Oriented Design of S3
A Few Words About Buckets
A Few Words About Objects
What If S3 Was a Standalone Library?
Resources
HTTP Response Codes
An S3 Client
The Bucket List
The Bucket
The S3 Object
Request Signing and Access Control
Signing a URI
Setting Access Policy
Using the S3 Client Library
Clients Made Transparent with ActiveResource
Creating a Simple Service
An ActiveResource Client
A Python Client for the Simple Service
Parting Words
Chapter 4. The Resource-Oriented Architecture
Resource-Oriented What Now?
What’s a Resource?
URIs
URIs Should Be Descriptive
The Relationship Between URIs and Resources
Addressability
Statelessness
Application State Versus Resource State
Representations
Deciding Between Representations
Links and Connectedness
The Uniform Interface
GET, PUT, and DELETE
HEAD and OPTIONS
POST
Creating subordinate resources
Appending to the resource state
Overloaded POST: The not-so-uniform interface
Safety and Idempotence
Safety
Idempotence
Why safety and idempotence matter
Why the Uniform Interface Matters
That’s It!
Chapter 5. Designing Read-Only Resource-Oriented Services
Resource Design
Turning Requirements Into Read-Only Resources
Figure Out the Data Set
General Lessons
Split the Data Set into Resources
General Lessons
Name the Resources
Encode Hierarchy into Path Variables
No Hierarchy? Use Commas or Semicolons
Map URIs
Scale
Algorithmic Resource? Use Query Variables
URI Recap
Design Your Representations
The Representation Talks About the State of the Resource
The Representation Links to Other States
Representing the List of Planets
Representing Maps and Points on Maps
Representing the Map Tiles
Representing Planets and Other Places
Representing Lists of Search Results
Link the Resources to Each Other
The HTTP Response
What’s Supposed to Happen?
Conditional HTTP GET
What Might Go Wrong?
Conclusion
Chapter 6. Designing Read/Write Resource-Oriented Services
User Accounts as Resources
Why Should User Accounts Be Resources?
Authentication, Authorization, Privacy, and Trust
Turning Requirements into Read/Write Resources
Figure Out the Data Set
Split the Data Set into Resources
Name the Resources with URIs
Expose a Subset of the Uniform Interface
Design the Representation(s) Accepted from the Client
Design the Representation(s) to Be Served to the Client
Link This Resource to Existing Resources
What’s Supposed to Happen?
What Might Go Wrong?
Custom Places
Figure Out the Data Set
Split the Data Set into Resources
Name the Resources with URIs
Expose a Subset of the Uniform Interface
Design the Representation(s) Accepted from the Client
Design the Representation(s) Served to the Client
Link This Resource to Existing Resources
What’s Supposed to Happen?
What Might Go Wrong?
A Look Back at the Map Service
Chapter 7. A Service Implementation
A Social Bookmarking Web Service
Figuring Out the Data Set
Resource Design
REST in Rails
The User Controller
The Bookmarks Controller
The User Tags Controller
The Calendar Controller
The URI Controller
The Recent Bookmarks Controller
The Bundles Controller
The Leftovers
Remodeling the REST Way
Implementation: The routes.rb File
Design the Representation(s) Accepted from the Client
Design the Representation(s) Served to the Client
Connect Resources to Each Other
What’s Supposed to Happen?
What Might Go Wrong?
Controller Code
What Rails Doesn’t Do
Conditional GET
param[:id] for things that aren’t IDs
The ApplicationController
The UsersController
The BookmarksController
The TagsController
The Lesser Controllers
The CalendarController
The RecentController
The UrisController
Model Code
The User Model
The Bookmark Model
What Does the Client Need to Know?
Natural-Language Service Description
Description Through Standardization
Hypermedia Descriptions
Chapter 8. REST and ROA Best Practices
Resource-Oriented Basics
The Generic ROA Procedure
Addressability
Representations Should Be Addressable
State and Statelessness
Connectedness
The Uniform Interface
Safety and Idempotence
New Resources: PUT Versus POST
Overloading POST
This Stuff Matters
Why Addressability Matters
Why Statelessness Matters
Why the Uniform Interface Matters
Why Connectedness Matters
A terrifying example
Resource Design
Relationships Between Resources
Asynchronous Operations
Batch Operations
Transactions
When In Doubt, Make It a Resource
URI Design
Outgoing Representations
Incoming Representations
Service Versioning
Permanent URIs Versus Readable URIs
Standard Features of HTTP
Authentication and Authorization
Basic authentication
Digest authentication
WSSE username token
Compression
Conditional GET
Caching
Please cache
Thank you for not caching
Default caching rules
Look-Before-You-Leap Requests
Partial GET
Faking PUT and DELETE
The Trouble with Cookies
Why Should a User Trust the HTTP Client?
Applications with a Web Interface
Applications with No Web Interface
What Problem Does this Solve?
Chapter 9. The Building Blocks of Services
Representation Formats
XHTML
XHTML with Microformats
Atom
OpenSearch
SVG
Form-Encoded Key-Value Pairs
JSON
RDF and RDFa
Framework-Specific Serialization Formats
Ad Hoc XHTML
Other XML Standards and Ad Hoc Vocabularies
Encoding Issues
XML and HTTP: Battle of the encodings
The character encoding of a JSON document
Prepackaged Control Flows
General Rules
Database-Backed Control Flow
GET
PUT
POST for creating a new resource
POST for appending to a resource
DELETE
The Atom Publishing Protocol
Collections
Members
Service document
Category documents
Binary documents as APP members
Summary
GData
Querying collections
Data extensions
POST Once Exactly
Hypermedia Technologies
URI Templates
XHTML 4
XHTML 4 links
XHTML 4 forms
Shortcomings of XHTML 4
XHTML 5
WADL
Describing a del.icio.us resource
Describing an APP collection
Is WADL evil?
Chapter 10. The Resource-Oriented Architecture Versus Big Web Services
What Problems Are Big Web Services Trying to Solve?
SOAP
The Resource-Oriented Alternative
WSDL
The Resource-Oriented Alternative
UDDI
The Resource-Oriented Alternative
Security
The Resource-Oriented Alternative
Reliable Messaging
The Resource-Oriented Alternative
Transactions
The Resource-Oriented Alternative
BPEL, ESB, and SOA
Conclusion
Chapter 11. Ajax Applications as REST Clients
From AJAX to Ajax
The Ajax Architecture
A del.icio.us Example
The Advantages of Ajax
The Disadvantages of Ajax
REST Goes Better
Making the Request
Handling the Response
JSON
Don’t Bogart the Benefits of REST
Cross-Browser Issues and Ajax Libraries
Prototype
Dojo
Subverting the Browser Security Model
Request Proxying
JavaScript on Demand
Dynamically writing the script tag
Library support
Chapter 12. Frameworks for RESTful Services
Ruby on Rails
Routing
Resources, Controllers, and Views
Outgoing Representations
Incoming Representations
Web Applications as Web Services
The Rails/ROA Design Procedure
Restlet
Basic Concepts
Writing Restlet Clients
Writing Restlet Services
Resource and URI design
Request handling and representations
Compiling, running, and testing
Conclusion
Django
Create the Data Model
Define Resources and Give Them URIs
Implement Resources as Django Views
The bookmark list view
The bookmark detail view
Further directions
Conclusion
Appendix A. Some Resources for REST and Some RESTful Resources
Standards and Guides
HTTP and URI
RESTful Architectures
Hypermedia Formats
Frameworks for RESTful Development
Weblogs on REST
Services You Can Use
Service Directories
Read-Only Services
Read/Write Services
Appendix B. The HTTP Response Code Top 42
Three to Seven Status Codes: The Bare Minimum
1xx: Meta
100 (“Continue”)
101 (“Switching Protocols”)
2xx: Success
200 (“OK”)
201 (“Created”)
202 (“Accepted”)
203 (“Non-Authoritative Information”)
204 (“No Content”)
205 (“Reset Content”)
206 (“Partial Content”)
207 (“Multi-Status”)
3xx: Redirection
300 (“Multiple Choices”)
301 (“Moved Permanently”)
302 (“Found”)
303 (“See Other”)
304 (“Not Modified”)
305 (“Use Proxy”)
306: Unused
307 (“Temporary Redirect”)
4xx: Client-Side Error
400 (“Bad Request”)
401 (“Unauthorized”)
402 (“Payment Required”)
403 (“Forbidden”)
404 (“Not Found”)
405 (“Method Not Allowed”)
406 (“Not Acceptable”)
407 (“Proxy Authentication Required”)
408 (“Request Timeout”)
409 (“Conflict”)
410 (“Gone”)
411 (“Length Required”)
412 (“Precondition Failed”)
413 (“Request Entity Too Large”)
414 (“Request-URI Too Long”)
415 (“Unsupported Media Type”)
416 (“Requested Range Not Satisfiable”)
417 (“Expectation Failed”)
5xx: Server-Side Error
500 (“Internal Server Error”)
501 (“Not Implemented”)
502 (“Bad Gateway”)
503 (“Service Unavailable”)
504 (“Gateway Timeout”)
505 (“HTTP Version Not Supported”)
Appendix C. The HTTP Header Top Infinity
Standard Headers
Accept
Accept-Charset
Accept-Encoding
Accept-Language
Accept-Ranges
Age
Allow
Authorization
Cache-Control
Connection
Content-Encoding
Content-Language
Content-Length
Content-Location
Content-MD5
Content-Range
Content-Type
Date
ETag
Expect
Expires
From
Host
If-Match
If-Modified-Since
If-None-Match
If-Range
If-Unmodified-Since
Last-Modified
Location
Max-Forwards
Pragma
Proxy-Authenticate
Proxy-Authorization
Range
Referer
Retry-After
TE
Trailer
Transfer-Encoding
Upgrade
User-Agent
Vary
Via
Warning
WWW-Authenticate
Nonstandard Headers
Cookie
POE
POE-Links
Set-Cookie
Slug
X-HTTP-Method-Override
X-WSSE
Index
The book hasn't received reviews yet.